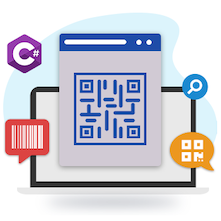
Barcodes are essential for product identification, providing machine-readable representations of data. Common formats like Code128, QR, DataMatrix, and Aztec are widely used across various industries. In this guide, you’ll learn how to generate barcodes in C# and customize their designs effortlessly using the powerful Aspose.BarCode Plugin.
Table of Contents
- C# Barcode Generator API
- How to Generate a Barcode using C#
- How to Generate a QR Barcode using C#
- Customize the Appearance of a Barcode in C#
- Add Caption in Barcodes using C#
C# Barcode API - Free Download
The Aspose.BarCode for .NET library is a comprehensive API designed for barcode generation and scanning. It allows you to create and read a variety of barcode symbologies, including:
- Code128
- Code11
- Code39
- QR
- DataMatrix
- EAN13
- EAN8
- ITF14
- PDF417
- and many more.
You can download the API for free or install it directly in your .NET application using NuGet:
PM> Install-Package Aspose.Barcode
How to Generate a Barcode using C#
Generating a barcode with the Aspose Plugin is straightforward and efficient. Follow these steps to create a barcode using C#:
- Instantiate the BarcodeGenerator class, specifying the desired barcode type and text in the constructor.
- Configure the barcode’s features, such as resolution and size.
- Generate the barcode using the BarcodeGenerator.Save(String) method.
Here’s a code sample demonstrating how to generate a barcode using C#:
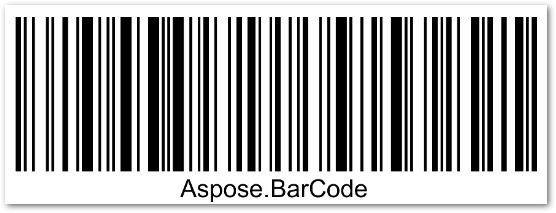
How to Generate a QR Barcode using C#
Creating a QR barcode follows a similar process to generating other barcode types. Here’s how to do it:
- Instantiate the BarcodeGenerator class, specifying the barcode type as EncodeTypes.QR.
- Generate the barcode using the BarcodeGenerator.Save(String) method.
Here’s a code sample for generating a QR barcode using C#:
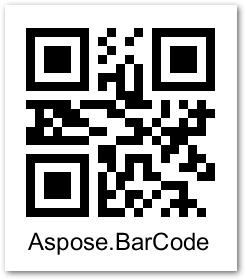
Customize the Appearance of a Barcode in C#
Customizing the appearance of a barcode is easy with the Aspose Plugin. You can modify its font, foreground color, background color, and text color. Here are the steps:
- Instantiate the BarcodeGenerator class.
- Adjust the barcode’s appearance using the BarcodeGenerator.Parameters properties, such as BarcodeGenerator.Parameters.BackColor.
- Generate the barcode using the BarcodeGenerator.Save(String) method.
Here’s a code sample for generating a customized Aztec barcode using C#:
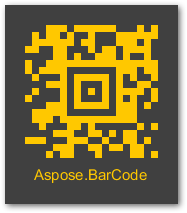
Add Caption in Barcodes using C#
Incorporating captions into barcodes can provide additional context, and the Aspose Plugin makes this process seamless. Here’s how to add a caption:
- Instantiate the BarcodeGenerator class.
- Set the barcode’s text and type in the constructor of BarcodeGenerator.
- Utilize the CaptionAbove or CaptionBelow properties to configure the caption.
- Save the barcode using the BarcodeGenerator.Save(String) method.
Here’s a code sample for adding a caption to a barcode using C#:
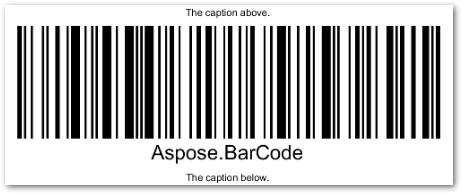
Conclusion
In this article, you learned how to programmatically generate barcodes using C#. You also explored how to customize their appearance and add captions. For more detailed information, visit the Aspose.BarCode documentation to enhance your barcode generation capabilities.