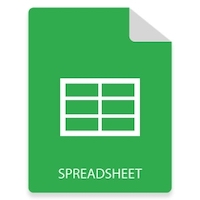
Excel spreadsheets are a prevalent format for storing and organizing data, making them invaluable for businesses and individuals alike. Whether you’re analyzing financial data, generating reports, or manipulating datasets, being able to work with Excel files in your C# applications is often essential.
Aspose.Cells for .NET is a powerful library that simplifies Excel file manipulation in C#. In this blog post, we will explore how to read Excel files using C# in a .NET application, providing a step-by-step guide to help you get started.
Table of Contents
- C# API to Read Excel Files
- Read Data from an Excel File in C#
- Read a Specific Worksheet in an Excel File
C# API to Read Excel Files
To read data from Excel sheets, we will utilize Aspose.Cells for .NET. This feature-rich API allows you to create and manipulate Excel files with ease. You can download it from the downloads section or install it via NuGet.
PM> Install-Package Aspose.Cells
Read Data from an Excel File in C#
In Excel files, data is stored in cells, each identified by its name (e.g., A1, B3) or by row and column indices. A collection of these cells forms a worksheet, and an Excel file may contain one or multiple worksheets. Together, all the worksheets make up a workbook. Aspose.Cells for .NET adheres to these naming conventions for Excel file manipulation.
Here are the steps to read an Excel file and extract data in C#:
- Load the Excel file using the Workbook class.
- Create an instance of the WorksheetCollection class and reference the worksheets using Workbook.Worksheets.
- Loop through all the worksheets in the collection:
- Get a reference to each worksheet in a Worksheet object.
- Count the data rows and columns in the worksheet.
- Loop through rows and nested loop through columns.
- Read data from each cell using the Worksheet.Cells[i, j].Value property.
Here’s a code sample that demonstrates how to read an Excel file in C#:
After running the above code sample, the console output will display the data extracted from the Excel file:
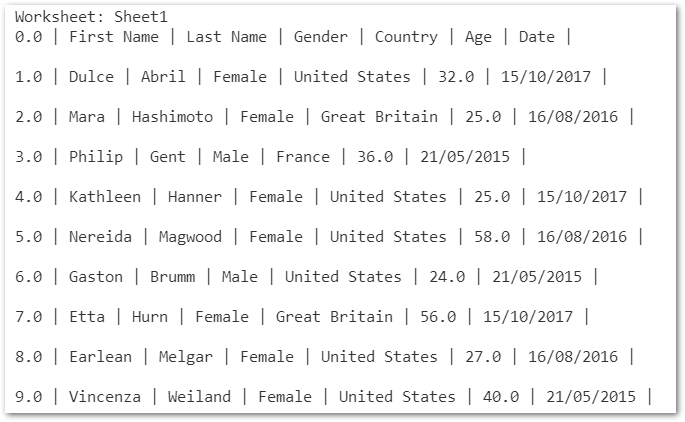
Reading an Excel File in C#
Read a Specific Excel Sheet
If you only need to read a specific worksheet in the Excel file, you can follow these steps:
- Load the Excel file using the Workbook class.
- Get a reference to the desired worksheet using Workbook.Worksheets[index].
- Count the data rows and columns in the worksheet.
- Loop through rows and nested loop through columns.
- Read data from each cell using the Worksheet.Cells[i, j].Value property.
Here’s a code sample for reading data from a specific worksheet in C#:
Get a Free API to Read Excel Data
You can use Aspose.Cells for .NET to read Excel files without evaluation limitations by obtaining a free temporary license.
Conclusion
Reading Excel files in C# using Aspose.Cells for .NET is a straightforward and efficient process. This powerful API provides a comprehensive set of features for handling all aspects of Excel file manipulation in your .NET applications. Whether you need to extract data, format sheets, or perform advanced tasks, Aspose.Cells makes the process seamless and helps you achieve your Excel-related goals effortlessly.
Explore more features of Aspose.Cells for .NET through the documentation. If you have any questions, feel free to reach out via our forum.