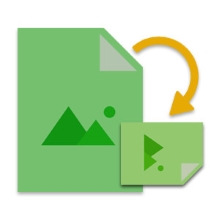
If you’re developing applications that require robust image editing capabilities, the $99 Aspose.Imaging Image Rotate & Flip Plugin is an excellent choice. This powerful .NET Plugin simplifies the process of flipping and rotating images, making it ideal for tasks such as user uploads, graphic design, or batch processing. With Aspose.Imaging for .NET, you can easily perform horizontal and vertical flips, as well as combine these actions with rotation.
In this guide, you will discover how to flip images programmatically in C#, including methods for horizontal flipping, vertical flipping, and combining flips with rotations.
Topics Covered
C# API to Flip Images
The Aspose.Imaging for .NET library offers a comprehensive API for image manipulation. With the RotateFlip method, you can easily apply various transformations to a wide range of image formats, including both vertical and horizontal flips. You can install this image processing API via NuGet or download the assembly files for integration into your project.
PM> Install-Package Aspose.Imaging
Steps to Flip an Image
To flip an image using the Aspose.Imaging library, follow these straightforward steps:
- Load the Image: Start by loading the image file you want to modify.
- Apply the Flip: Use the
RotateFlip
method to specify the flipping direction (horizontal or vertical). - Save the Image: Finally, save the modified image in your desired format.
Flip an Image
Here’s a simple example demonstrating how to flip an image horizontally in C#:
using Aspose.Imaging;
using Aspose.Imaging.ImageOptions;
public void FlipImageHorizontally(string inputPath, string outputPath)
{
using (Image image = Image.Load(inputPath))
{
image.RotateFlip(RotateFlipType.RotateNoneFlipX);
image.Save(outputPath, new PngOptions());
}
}
Rotate and Flip an Image
You can also combine rotation with flipping. Here’s how to do both in one go:
public void RotateAndFlipImage(string inputPath, string outputPath)
{
using (Image image = Image.Load(inputPath))
{
image.RotateFlip(RotateFlipType.Rotate90FlipY);
image.Save(outputPath, new PngOptions());
}
}
By following these steps, you can easily manipulate images in your .NET applications using the powerful features of the Aspose.Imaging library. Whether for personal projects or professional applications, flipping and rotating images has never been easier!
For more advanced image processing tasks, consider exploring the Image Conversion API to convert JPEG images to DICOM using C#, or check out the best ODG to SVG converter for .NET to further enhance your application’s capabilities.