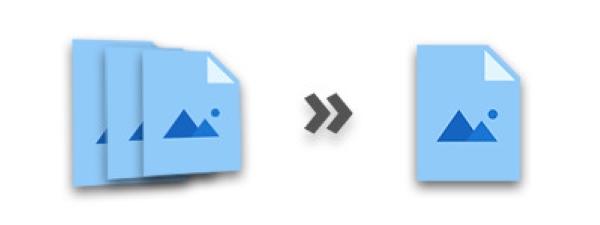
The Aspose.Imaging Image Merger Plugin for .NET makes it incredibly simple to programmatically combine images either horizontally or vertically, all while preserving image quality. This powerful plugin is designed specifically for developers and supports a wide variety of formats, allowing you to create stunning photo collages effortlessly. In this guide, you will learn how to merge or combine multiple images programmatically in C#.
Topics Covered:
C# API to Merge Images
To combine images in C# programmatically, we will utilize Aspose.Imaging for .NET, a robust .NET image processing library designed for high-performance image merging. This API allows for precise image manipulations and supports a wide range of formats. You can easily integrate the API into your .NET projects by downloading the plugin or installing it via NuGet:
PM> Install-Package Aspose.Imaging
Merge Multiple Images in C#
There are two primary methods to merge images into a single file: vertical image concatenation and horizontal image concatenation. In vertical concatenation, images are stacked one above the other, while in horizontal concatenation, images are placed side by side. The following sections will guide you through both methods with practical code samples.
C# Merge Images Vertically
To merge images vertically in C#, follow these steps:
- Specify the paths of the images in a string array.
- Create a list of Size to store the dimensions of each image.
- Calculate the total height and width of the resulting image.
- Create an object of the StreamSource class, initializing it with a new MemoryStream.
- Create an object of JpegOptions class and configure its options.
- Instantiate a JpegImage for the new image, initializing it with the specified JpegOptions and calculated dimensions.
- Iterate through the list of images, loading each into a RasterImage object.
- Create a Rectangle for each image and add it to the new image using the JpegImage.SaveArgb32Pixels() method.
- Increment the stitched height during each iteration.
- Finally, save the new image using the JpegImage.Save(string) method.
Here’s a code sample demonstrating how to merge images vertically in C#:
C# Merge Images Horizontally
To combine images horizontally in C#, follow these steps:
- Specify the paths of the images in a string array.
- Create a list of Size to store the dimensions of each image.
- Calculate the total height and width of the resulting image.
- Create a new source using FileCreateSource(String, Boolean) and initialize it with the file’s path.
- Create an object of JpegOptions class and configure its options.
- Instantiate a JpegImage for the new image, initializing it with the specified JpegOptions and calculated dimensions.
- Iterate through the list of images, loading each into a RasterImage object.
- Create a Rectangle for each image and add it to the new image using the JpegImage.SaveArgb32Pixels() method.
- Increment the stitched width during each iteration.
- Once completed, save the new image using the JpegImage.Save(string) method.
Here’s a code sample demonstrating how to merge multiple images horizontally in C#:
C# Image Merging API - Get a Free License
You can obtain a free temporary license to merge images without evaluation restrictions.
Conclusion
In this article, you have learned how to programmatically merge images into a single file using C#. The provided code samples illustrate how to combine images programmatically both vertically and horizontally. For further exploration of the .NET image processing library for merging, refer to the documentation. Additionally, feel free to share your queries with us via our forum.