Are you looking for a quick and efficient way to generate rich PDF files in C#? If so, you’ve come to the right place! This article will guide you through the process of creating PDF files from scratch, making it easy to add various components to your documents.
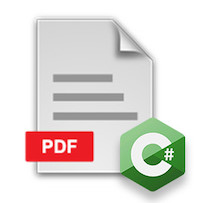
Automated generation and processing of PDF documents have become essential in many applications, from invoices to reports. In this guide, you will learn how to create PDF files in C# and how to insert text, images, tables, and other components into PDFs using C#.
Table of Contents
- C# PDF Library - Free Download
- Create PDF Files in C#
- Edit Existing PDF in C#
- Insert Image in PDF using C#
- Create a Table in PDF using C#
- Create a Form in PDF in C#
C# .NET PDF Library to Generate PDF
Aspose.PDF for .NET is a powerful PDF manipulation library that enables you to generate and process PDF files directly from your .NET applications. With this library, you can dynamically create various types of reports and business documents. Additionally, you can obtain a free license to start generating your desired PDF files without any limitations.
You can download the library as a DLL or install it directly from NuGet using the following command:
Install-Package Aspose.Pdf
Create PDF Files in C#
Let’s start by creating a simple PDF document that includes a text fragment. Follow these steps to create a PDF file using C#:
- Create an object of the Document class.
- Add a page to the document using the Document.Pages.Add() method.
- Create a new TextFragment object and set its text.
- Add the TextFragment to the Paragraphs collection of the page.
- Save the PDF file using the Document.Save(String) method.
Here’s a code sample demonstrating how to create a simple PDF file in C#:
For more complex PDF document creation, check out our guide on creating complex PDF documents.
How to Edit a PDF File in C#
Editing a PDF file is just as straightforward as creating one. To modify a PDF, simply load the file using the Document class, perform your desired operations, and save it. Here are the steps to modify a PDF:
- Create an object of the Document class and provide the path to the PDF file.
- Manipulate the pages or content of the document as needed.
- Save the document using the Document.Save() method.
Here’s a code sample to illustrate how to modify a PDF using C#:
Insert Image in PDF using C#
Next, let’s explore how to insert an image into your PDF document. Follow these steps:
- Create an object of the Document class to open a PDF document.
- Access the page where you want to add the image using the Page class.
- Add the image to the page’s Resources collection.
- Use the following operators to place the image on the page:
- GSave to save the current graphical state.
- ConcatenateMatrix to specify the placement of the image.
- Do to draw the image on the page.
- Finally, use the GRestore operator to restore the graphical state.
- Save the PDF file.
Here’s a code sample to show how to add an image to a PDF document using C#:
For more details, read our guide on inserting images in PDF.
Create a Table in PDF using C#
Tables are vital for organizing data in rows and columns within documents. Aspose.PDF for .NET makes it easy to create and insert tables in PDF documents. Here’s how to do it:
- Load the PDF file using the Document class.
- Initialize a table and define its columns and rows using the Table class.
- Set the table’s settings (e.g., borders).
- Populate the table by creating rows with the Table.Rows.Add() method.
- Add the table to the page using the Document.Pages[1].Paragraphs.Add(Table) method.
- Save the PDF file.
Here’s a code sample to create and add a table to a PDF document in C#:
For more information, check out our guide on creating tables in PDF.
Create a Form in PDF in C#
Forms in PDFs are essential for collecting data from users. You can insert various controls like textboxes, checkboxes, and radio buttons in PDF forms. The PDF format supports two types of forms: Acro forms and XFA forms (see details). Here’s how to create and add forms in a PDF:
- Load the PDF file using the Document class.
- Create form controls such as TextBoxField.
- Add the control to the form using the Document.Form.Add(textBoxField, 1) method.
- Save the PDF document.
Here’s a code sample to add forms to the PDF document using C#:
For further details, read our guide on creating forms in PDF.
Free C# PDF Library
You can obtain an absolutely free temporary license to generate PDF files without any limitations.
Free Online PDF Viewer and Editor
Aspose.PDF offers a free online web application that allows you to view PDFs and edit PDFs.
Conclusion
In this post, you have learned how to create PDF files from scratch using C#. Additionally, you now know how to insert various components such as text, images, tables, and forms into a PDF document programmatically. To further explore the capabilities of the PDF API, refer to the official documentation.