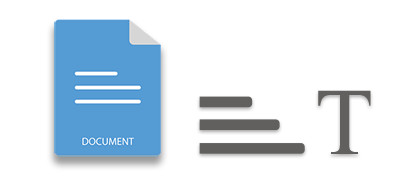
Microsoft Word documents are a staple for creating and sharing textual content. If you’re developing C# applications that interact with these documents, you might find yourself needing to extract text from them. This could be for purposes such as text analysis or extracting specific sections of a document to compile into a new one. In this blog post, we will dive into the methods for extracting text from Word documents in C#.
Table of Contents
- C# Library for Text Extraction
- Understanding Text Extraction in Word Documents
- Step-by-Step Guide to Extract Text from a Word Document
C# Library for Text Extraction
Aspose.Words for .NET is a powerful and user-friendly library designed for working with Word documents. It provides a comprehensive set of features, including text extraction, document creation, manipulation, and conversion. With Aspose.Words for .NET, developers can efficiently manage various aspects of Word documents, making it an invaluable tool for your development needs.
To get started, download the library or install it directly from NuGet using the following command in the package manager console:
PM> Install-Package Aspose.Words
Understanding Text Extraction in Word Documents
An MS Word document comprises various elements such as paragraphs, tables, and images. Consequently, the requirements for text extraction can differ based on the specific use case. You may need to extract text between paragraphs, bookmarks, comments, and more.
Each element in a Word document is represented as a node. Therefore, to effectively process a document, you will need to work with these nodes. Let’s explore how to extract text from Word documents in different scenarios.
Step-by-Step Guide to Extract Text from a Word Document
In this section, we will implement a C# text extractor for Word documents. The workflow for text extraction will involve the following steps:
- Define the nodes to include in the extraction process.
- Extract the content between the specified nodes (including or excluding the starting and ending nodes).
- Use the cloned extracted nodes to create a new Word document containing the extracted content.
Let’s create a method named ExtractContent that will accept nodes and other parameters to perform the text extraction. This method will parse the document and clone the nodes based on the following parameters:
- StartNode and EndNode: These define the starting and ending points for content extraction. They can be block-level (e.g., Paragraph, Table) or inline-level nodes (e.g., Run, FieldStart, BookmarkStart).
- For fields, pass the corresponding FieldStart object.
- For bookmarks, use BookmarkStart and BookmarkEnd nodes.
- For comments, employ CommentRangeStart and CommentRangeEnd nodes.
- IsInclusive: This parameter determines whether the markers are included in the extraction. If set to false and the same or consecutive nodes are provided, an empty list will be returned.
Here is the complete implementation of the ExtractContent method to extract content between the specified nodes:
Additionally, some helper methods are required by the ExtractContent method to facilitate the text extraction operation:
Now that we have our methods ready, we can proceed to extract text from a Word document.
Extracting Text Between Paragraphs of a Word Document
To extract content between two paragraphs in a Word DOCX document, follow these steps:
- Load the Word document using the Document class.
- Get references to the starting and ending paragraphs using the Document.FirstSection.Body.GetChild(NodeType.PARAGRAPH, int, boolean) method.
- Call the ExtractContent(startPara, endPara, True) method to extract the nodes into an object.
- Use the GenerateDocument(Document, extractedNodes) helper method to create a document with the extracted content.
- Save the new document using the Document.Save(string) method.
Here’s a code sample demonstrating how to extract text between the 7th and 11th paragraphs in a Word document:
Extracting Text Between Different Types of Nodes
You can also extract content between different types of nodes. For example, let’s extract content between a paragraph and a table and save it into a new Word document. The steps are as follows:
- Load the Word document using the Document class.
- Get references to the starting and ending nodes using the Document.FirstSection.Body.GetChild(NodeType, int, boolean) method.
- Call ExtractContent(startPara, endPara, True) to extract the nodes into an object.
- Use the GenerateDocument(Document, extractedNodes) helper method to create a document with the extracted content.
- Save the new document using Document.Save(string).
Here’s the code sample for extracting text between a paragraph and a table in C#:
Extracting Text Based on Styles
To extract content between paragraphs based on styles, follow these steps. For this demonstration, we will extract content between the first “Heading 1” and the first “Heading 3” in the Word document:
- Load the Word document using the Document class.
- Extract paragraphs into an object using the ParagraphsByStyleName(Document, “Heading 1”) helper method.
- Extract paragraphs into another object using ParagraphsByStyleName(Document, “Heading 3”).
- Call ExtractContent(startPara, endPara, True) with the first elements from both paragraph arrays.
- Use the GenerateDocument(Document, extractedNodes) helper method to create a document with the extracted content.
- Save the new document using Document.Save(string).
Here’s a code sample to extract content between paragraphs based on styles:
Read More About Text Extraction
Explore additional scenarios for extracting text from Word documents through this documentation article.
Get a Free Word Text Extractor Library
You can obtain a free temporary license to extract text without evaluation limitations.
Conclusion
Aspose.Words for .NET is a versatile library that streamlines the process of extracting text from Word documents in C#. With its extensive features and user-friendly API, you can efficiently work with Word documents and automate various text extraction scenarios. Whether you’re developing applications that require Word document processing or simply extracting text, Aspose.Words for .NET is an essential tool for developers.
To explore more features of Aspose.Words for .NET, check out the documentation. If you have any questions, feel free to reach out via our forum.
See Also
Tip: You may want to check out the Aspose PowerPoint to Word Converter, which demonstrates the popular process of converting presentations to Word documents.