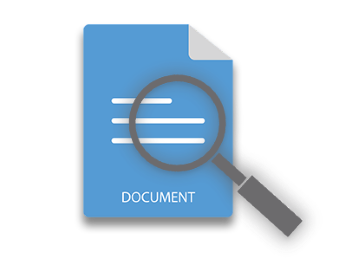
Introduction
Replacing text in Word documents is a crucial task for document editing, automation, and content updates. In this guide, we will explore how to find and replace text in Word documents (DOCX, DOC) in C# using Aspose.Words for .NET. This powerful library simplifies the automation of document editing tasks.
Why Automate Text Replacement in Word?
Automating text replacement offers several advantages:
- Quickly modify large Word documents with ease.
- Standardize content updates across multiple documents efficiently.
- Utilize regex-based searching for advanced text pattern matching.
Table of Contents
- Setting Up Word Text Replacement in C#
- Finding and Replacing Text in Word
- Using Regex to Replace Text
- Replacing Text in Headers and Footers
- Batch Process Multiple Word Files
- Getting a Free API License
- Conclusion and Additional Resources
1. Setting Up Word Text Replacement in C#
To perform text replacement in Word documents, we will utilize Aspose.Words for .NET. This library provides:
- Automated text find-and-replace for various formats including DOCX and DOC.
- Support for regex-based replacements for complex search scenarios.
- Efficient processing capabilities for large Word documents.
Installation
You can easily install Aspose.Words via NuGet with the following command:
PM> Install-Package Aspose.Words
Alternatively, you can download the DLL from the Aspose Downloads Page.
2. Finding and Replacing Text in Word
To replace text in a Word document programmatically, follow these steps:
- Load the Word file using the
Document
class. - Define FindReplaceOptions for text replacement.
- Execute the text replacement across the document.
Code Example
Here’s a simple code snippet to demonstrate the process:
// Load the document
Document doc = new Document("input.docx");
// Create a Find and Replace options object
FindReplaceOptions options = new FindReplaceOptions();
// Perform the find and replace operation
doc.Range.Replace("old text", "new text", options);
// Save the modified document
doc.Save("output.docx");
This method effectively automates text updates in Word files.
3. Using Regex to Replace Text
For pattern-based text replacement, leverage regular expressions as shown below:
FindReplaceOptions options = new FindReplaceOptions();
doc.Range.Replace(new Regex(@"Example"), "Updated Text", options);
This approach provides advanced text search-and-replace functionality.
4. Replacing Text in Headers and Footers
To modify headers and footers in Word documents, use the following code:
foreach (Section section in doc.Sections)
{
HeaderFooter header = section.HeadersFooters[HeaderFooterType.HeaderPrimary];
if (header != null)
{
header.Range.Replace("Old Header", "New Header", options);
}
}
This ensures content updates across all sections of the document.
5. Batch Process Multiple Word Files
To find and replace text across multiple Word documents, you can loop through a folder like this:
string[] files = Directory.GetFiles("input_docs", "*.docx");
foreach (string file in files)
{
Document doc = new Document(file);
doc.Range.Replace("Placeholder", "New Content", options);
doc.Save(file);
}
This method automates bulk text updates across numerous files.
6. Getting a Free API License
To unlock the full features of Aspose.Words, request a free temporary license.
For comprehensive documentation, visit the Aspose.Words Guide or engage with the community on the Aspose forum.
7. Conclusion and Additional Resources
Summary
In this guide, we covered:
✅ How to find and replace text in Word using C#
✅ Using regex for advanced text replacement
✅ Modifying headers and footers dynamically
✅ Batch processing multiple Word files
With Aspose.Words for .NET, you can efficiently automate text manipulation in Word documents. Start enhancing your document editing workflows today, and consider the Aspose Plugin for just $99 to unlock even more capabilities!