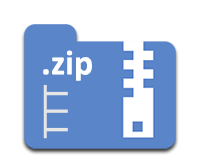
Introduction
Managing ZIP files can be challenging, especially when they contain nested archives. This guide will walk you through the process of flattening ZIP structures by extracting all inner ZIP archives into a single archive using Aspose.ZIP for .NET.
Why Create a Flat ZIP?
Creating a flat ZIP archive offers several advantages:
- Simplifies ZIP file management: Reduces complexity when handling multiple files.
- Extracts all nested ZIP contents into one archive: Consolidates files for easier access.
- Reduces redundant folder structures: Streamlines the organization of files.
Table of Contents
- Setting Up ZIP Archive Processing in C#
- Steps to Create a Flat ZIP Archive
- Saving the Flattened ZIP File
- Batch Processing Multiple ZIP Archives
- Getting a Free API License
- Conclusion and Additional Resources
1. Setting Up ZIP Archive Processing in C#
To effectively process nested ZIP archives, we will utilize Aspose.ZIP for .NET. This powerful library provides:
- Automated ZIP extraction and re-compression.
- Support for multiple archive formats: ZIP, TAR, GZip, and more.
- Efficient processing of large archives.
Installation
You can easily install the library via NuGet:
PM> Install-Package Aspose.Zip
Alternatively, you can download the DLL from the Aspose Downloads Page.
2. Steps to Create a Flat ZIP Archive
Consider the following nested ZIP structure:
parent.zip
├ first.txt
├ inner.zip
│ ├ file1.exe
│ └ data.bin
└ picture.gif
After flattening, all inner ZIP contents will be consolidated into the parent ZIP:
flattened.zip
├ first.txt
├ picture.gif
├ file1.exe
└ data.bin
Code Example
Here’s how you can automate the extraction of nested ZIP files:
// Load the parent ZIP file
using (Archive parentZip = new Archive("parent.zip"))
{
List<ArchiveEntry> toDelete = new List<ArchiveEntry>();
List<string> newEntryNames = new List<string>();
List<MemoryStream> newStreams = new List<MemoryStream>();
foreach (ArchiveEntry entry in parentZip.Entries)
{
if (entry.Name.EndsWith(".zip", StringComparison.OrdinalIgnoreCase))
{
toDelete.Add(entry);
using (MemoryStream zipStream = new MemoryStream())
{
entry.Open().CopyTo(zipStream);
zipStream.Position = 0;
using (Archive innerZip = new Archive(zipStream))
{
foreach (ArchiveEntry innerEntry in innerZip.Entries)
{
using (MemoryStream entryStream = new MemoryStream())
{
innerEntry.Open().CopyTo(entryStream);
newEntryNames.Add(innerEntry.Name);
newStreams.Add(entryStream);
}
}
}
}
}
}
foreach (var entry in toDelete)
parentZip.DeleteEntry(entry);
for (int i = 0; i < newEntryNames.Count; i++)
parentZip.CreateEntry(newEntryNames[i], newStreams[i]);
parentZip.Save("flattened.zip");
}
This method effectively automates nested ZIP file extraction, ensuring a smooth workflow.
3. Saving the Flattened ZIP File
Once you have extracted the nested ZIP contents, save the flattened ZIP file using the following line of code:
parentZip.Save("flattened.zip");
This ensures you have a single ZIP archive without nested folders, making file management much easier.
4. Batch Processing Multiple ZIP Archives
If you need to flatten multiple ZIP archives located in a specific folder, you can use the following code snippet:
string[] files = Directory.GetFiles("zip_folder", "*.zip");
foreach (string file in files)
{
using (Archive archive = new Archive(file))
{
archive.ExtractToDirectory("output_folder");
}
}
This method allows for automated bulk ZIP processing, saving you time and effort.
5. Getting a Free API License
To unlock the full features of Aspose.ZIP, you can request a free temporary license.
For comprehensive documentation, visit the Aspose.ZIP Guide. If you have any questions, feel free to engage with the community on the Aspose forum.
6. Conclusion and Additional Resources
Summary
In this guide, we covered:
✅ How to flatten ZIP files in C#
✅ Extracting nested ZIP archives
✅ Saving the final ZIP archive
✅ Processing multiple ZIP files at once
With Aspose.ZIP for .NET, you can efficiently extract and manage ZIP archives in your applications. Start optimizing your ZIP processing workflows today!